1. Initial events list
Since the Event component receives information like the date or title from the App
(parent) component, it is now the App component which will be responsible for storing
and managing list of events. Let's add an initial list of the events into the
app.component.ts
Now when we have our initial data, let's learn how to use for loop to dynamically generate all event from events[] array.
2. *ngFor
Angular allows us to variety of usefull directive. On on the most basic and common is ngFor
which is a implementation of for loop
.
ngFor
is designed work with the collections. Since we have collection of events let's see how to
use ngFor
directive in our code. Update app.component.html
with the following
code:
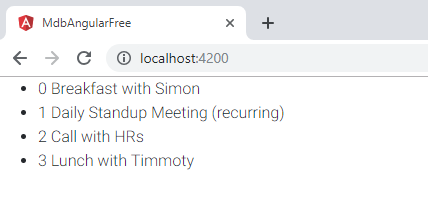
As you can see, it's very intuitive. We are browsing through events
collection and reffering
single instance as event
. We have also defined our autoincremented index as i
.
Of course names can be adjusted to our variables and convention i.e.:
We can also put more complex structure within our loop (i.e. surrounding div, headings etc.):
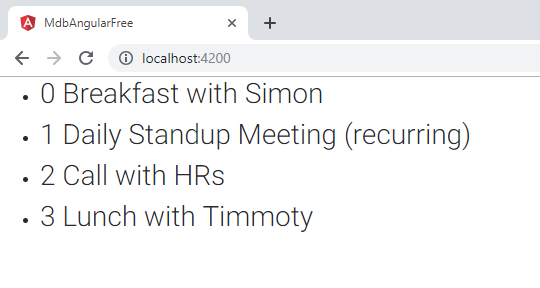
3. <ng-container>
As you probably noticed ngFor
directive is used inside the HTML tags like
<li>
.
This means that given tag will be duplicated as many times as our loop will iterate. Sometimes you may want
to use ngFor
loop outside the tag (don't want any element to be repeated).
Although it is impossible to use ngFor
directive outside the tag, there is another way. We can
use special Angular tag
:
This will not render any wraping element around our elements.
4. ngFor
vs *ngFor
You also may noticed that we are using a asterisk (*
) before the ngFor
directive.
It is related to so called templates
within Angular. We will learn more about them in a future
lesson. For now just remember that:
- Both
ngFor
and*ngFor
are valid Angular directives *ngFor
is simplified version ofngFor
which allows us to use it directly on elements like<li>
If you are curious what are the difference, have a look at following code. This:
is a shorter version of:
Therefore, before we will get to the lesson about Angular templates, keep in mind to rather use asterix if you wan't to avoid issues.
5. Using ngFor
to pass events
Now when we know how ngFor
works, let's use it to pass dynamically list of all events and render
them:
Note:
We skipped the index (iterator) part on purpose. We don't need to reffer to it within our loop, therefore we
haven't defined a variable for it.
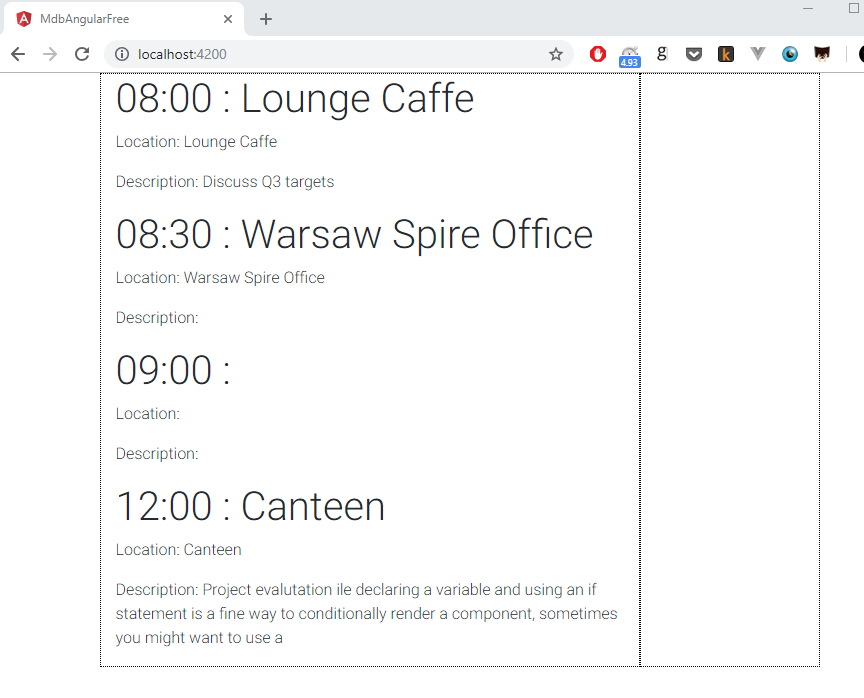