1. Download project files
Once we have our environment ready (i.e. npm installed). Let's download the working files. We can do it in two ways:
1.1 GIT repository
In order to download the working files, open a console and type:
1.2 ZIP file
If for some reason you don't want to use Git directly, you can simply download as zip file and extract it.
Info:
The working directory contains multiple folders for each section (getting-started, agenda-app) and inside
those are other folders called lesson-x where x is the number of the lesson. Each of the folders contains
the final code from a given lesson. In case of facing issues you can always refer to the corresponding
lesson folder, check final code and compare with yours to spot the difference.
2. Open project
Open a project in your favorite editor. I use and recommend Visual Studio Code (VSC) from Microsoft
In order to open a project using VSC:
- Open VSC
File
>Open Folder
- Navigate to
getting-started/start-here
folder - Click on
Select Folder
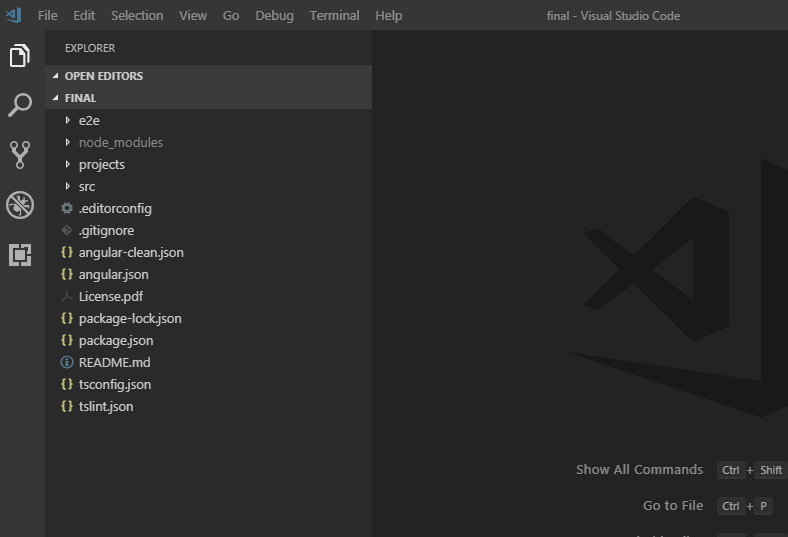
3. Start project
In order to start a project we will use Node Package Manager (npm)
. Make sure you have
npm installed in order to verify that you can open a default terminal/command line:
- Open Command line (below instruction how to open it for Windows, MacOS and Linux)
- Type
npm -v
and press Enter - Navigate to the project location using the command
cd
(the location on your computer will be different): - Install dependencies using the
npm install
command - Start the project using the
npm start
command - Open a web browser and navigate to http://localhost:4200
How to run command line?
Keyboard shortcut:
On your keyboard press: +
R . You will see Run
windows. Type cmd
and click OK or
press Enter
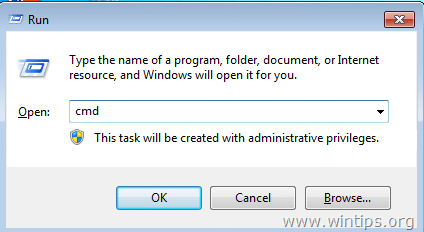
You will see a screen like below:
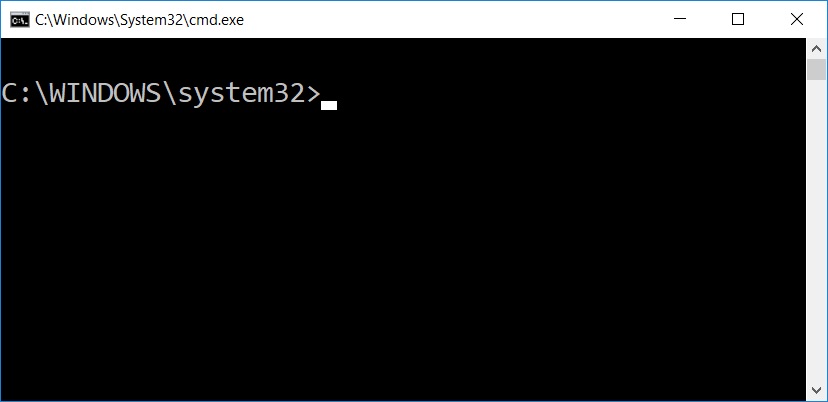
That's it. You can now use a command line, however, please keep in mind that Windows Command Line is - frankly speaking - a crappy tool. That's why we strongly encourage you to use some alternative such as GitBash (installation guide here). It has more Unix-like commands that are more user-friendly than built in windows commands.
How to run command line?
Keyboard shortcut:
Press cmd ⌘ + space to open Spotlight search, then type
terminal
and hit Enter ⏎
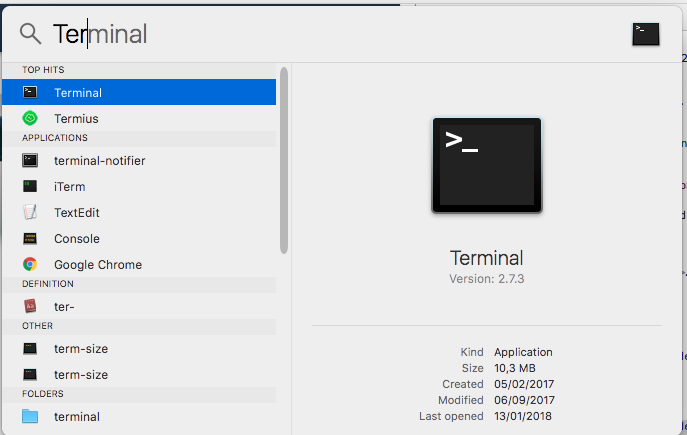
You will see a screen lke below:
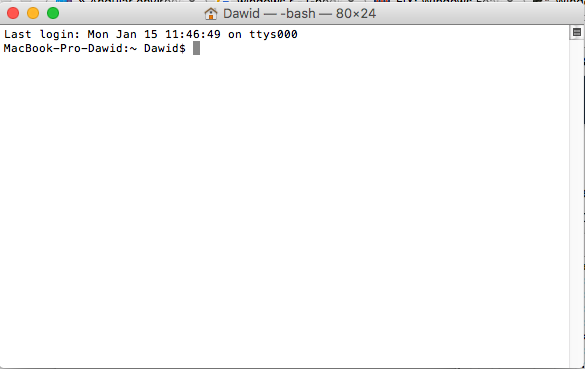
Unix unlike Windows or macOS, doesn't necessarily have a Graphic User Interface (GUI), therefore in some distributions like RedHat, CentOS, Debian, and Ubuntu Server the terminal will launch immediately after bootin up the machine.
In case you use a GUI, they may look different. However in most cases you they will have
Windows-like "Start" button which will open the search menu that allow you to find
terminal
app.
Sample start screen on Ubuntu:
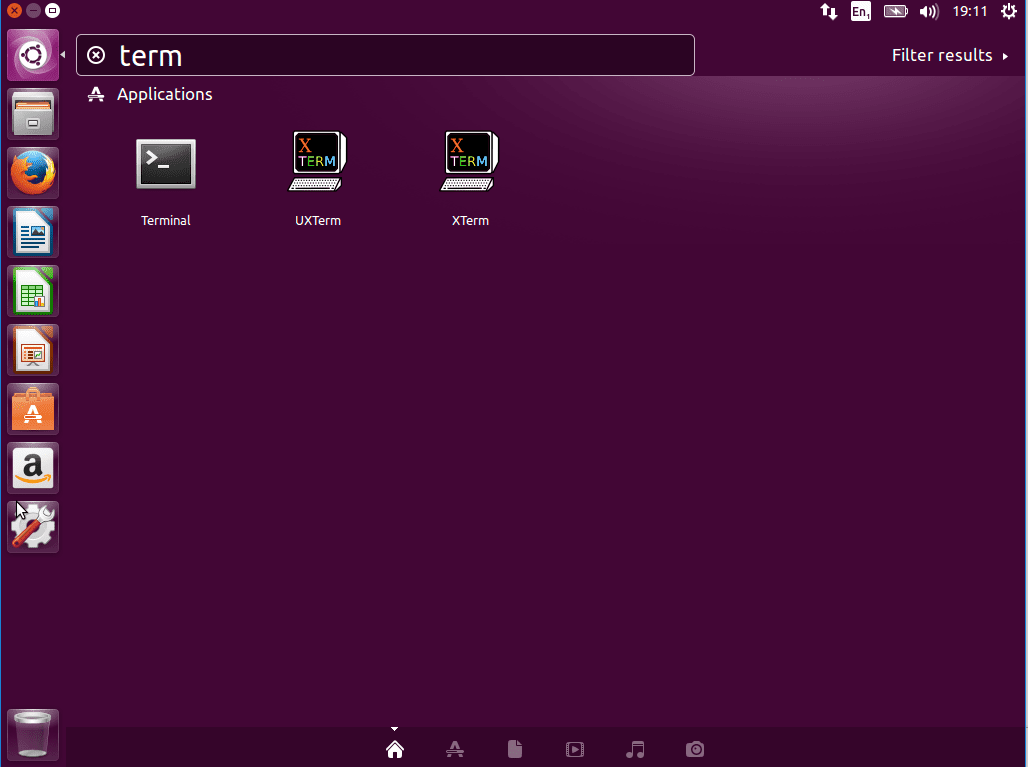
Also terminal windows and colors may vary depending on the distribution, - here is a sample Ubuntu terminal:
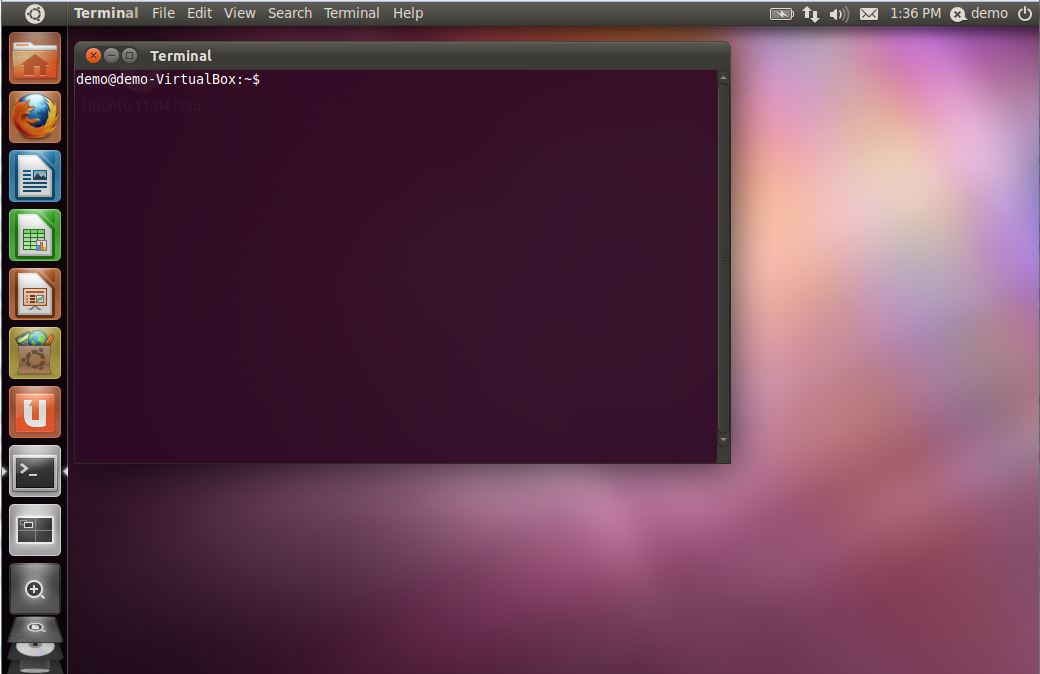
If you can see the version of npm we can start the project (go to the next step). Otherwise you have to install npm first. Here you can find the guide on how to do it.
cd C:\AngularApp\tutorial\AngularTutorialAgendaApp\getting-started\start-here>
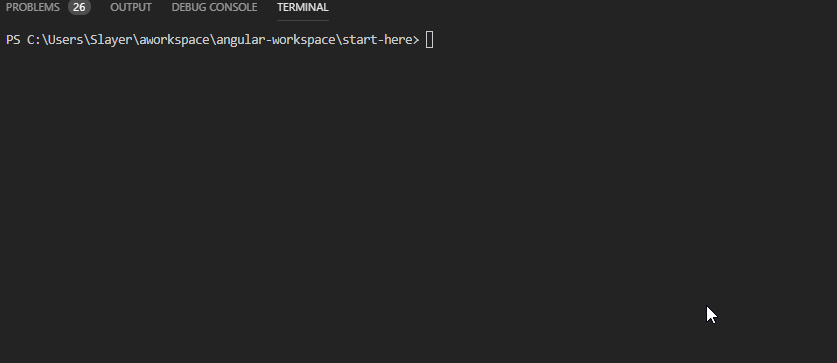
Note:
When you run npm start
command npm will invoke another command underneath:
ng serve
ng serve
is an Angular CLI command so you can use it directly to run your project instead
of npm start
4. Hello World!
- Open the
src/app/app.component.html
file. - Replace the content of the file with Hello World.
- Check the result in a browser:
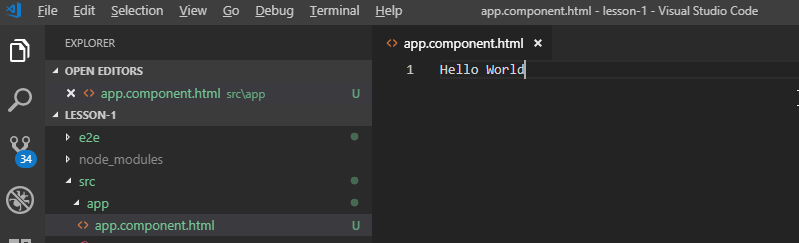
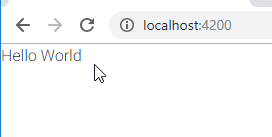
Voila! Our application is running. That was easy, wasn't it? Now let's have a closer look at our project structure to understand how Angular project works.
5. Project structure
Let's have a look at the files within our project. There are multiple folders and files and each is responsible for a variety of functionalities, however you don't have to know all of them, for now, I will introduce you to the key files and directories within our project.
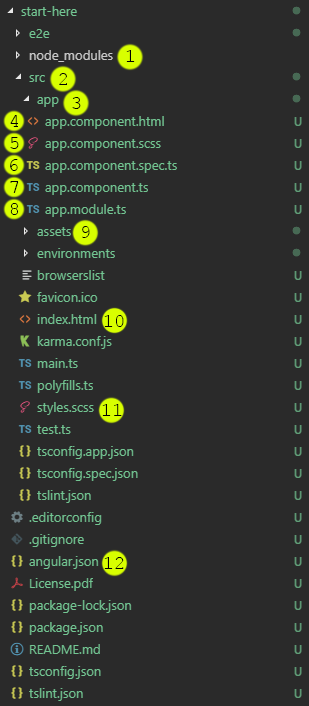
- The
node_modules
directory is where all of the libraries we need to build Angular are stored. - In the
src
directory, is placed the source code of your application. - The
app/
folder contains file related to our initial App component: - The
app.component.html
contains template of the component - The
app.component.scss
contains the CSS styles for the component - The
app.component.spec.ts
contains the tests of our component - The
app.component.ts
contains a TypeScript code (logic) of the component - The
app.module.ts
contains the configuration of the App module - In the
assets
directory, you place any static assets like images or icons. index.html
is the main file of our app.- The
styles.scss
contains the global styles for our application - The
angular.json
file contains the configuration of our app.
If we peek inside our index.html
file, we can see there’s a element
<app-root></app-root>, which will generate (render) our App component in the
following place.
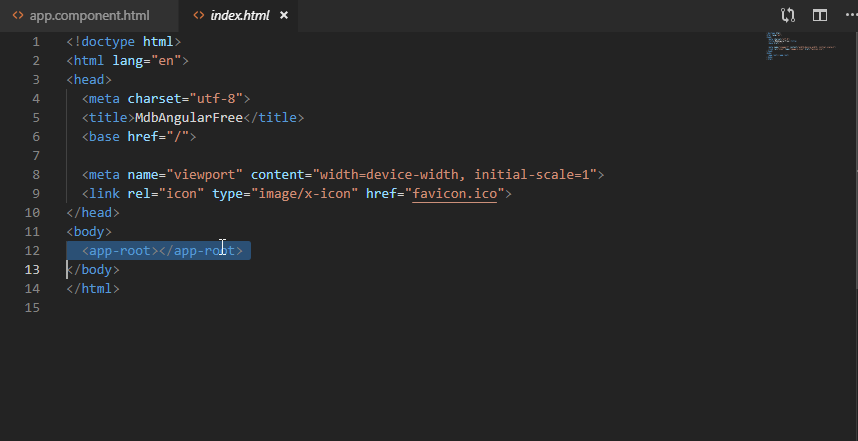
Note:
Within the project you can either use CSS, SASS, Less and Stylus. In case of CSS you would see the
style.css
file within your project. Since we are using SASS (the more powerfull CSS), there
is style.scss
file (note different extension).
The configuration whether project uses CSS or SASS is kept in angular.json
file. We will
learn how to switch between both in a future lesson. You can learn more about SASS here.
In the next lesson, we will learn how to use build sample components.
Important!
I am very happy that you reached this far. I would like to thank you and ask you for a small
favor. Please provide your comment on this lesson.
Your feedback is very important to us!. Please let us know
what you liked and what you didn't like to help us make later tutorials better and better!
It would be awesome if after each lesson you would be so kind and provide your comment on lesson. I would
appreciate that! Thanks!