1. Overview
Within the last lesson we went through the most important file within our project. Now we will focus on the components.
Among other advantages, Angular gained its position thanks to components-based architecture.
Components can be thought of as small pieces of an interface that are independent of each other. Imagine that you have a simple application with a list of items and a corresponding search box to retrieve the items by word matches. The box with listed names, the search box, and the main sheet where the other two boxes are placed are all considered separate components in Angular.
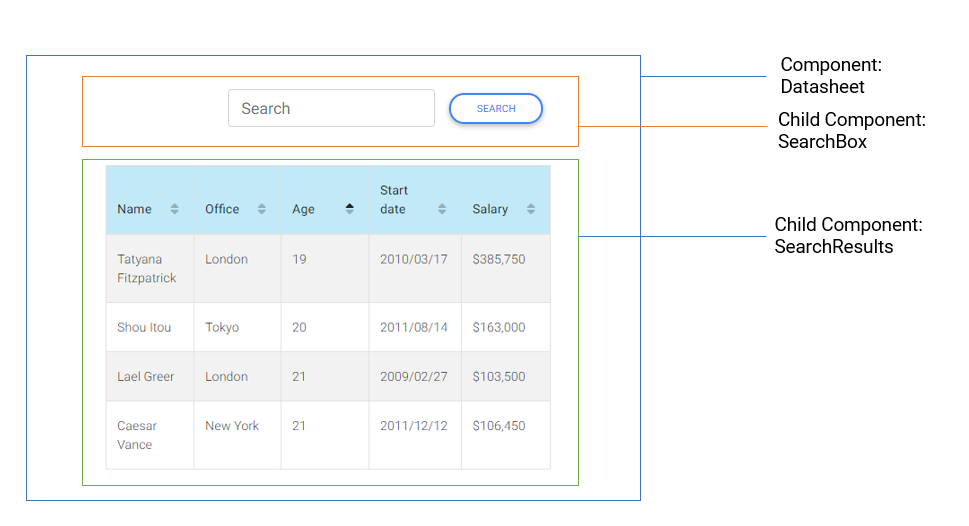
Components of a similar nature are well encapsulated, in other words, self-sufficient. Developers can reuse them across different parts of an application. This is particularly useful in enterprise-scope applications where different systems converge but may have many similar elements like search boxes, date pickers, sorting lists, etc.
Let's have a look how components are build within Angular code
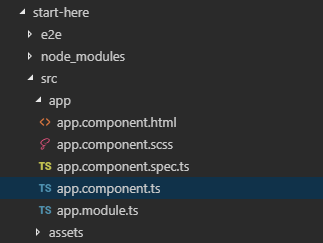
As you can see within a src/
location, there is a folder called app
containing a
few files starting with app.*
name inside. The app
represents App
component.
Usually components are organised within a folders (1 component = 1 folder). Each Angular project has to contain at least 1 component. Each component consist of few files:
2. Component logic (.ts
file)
Let's open file located in /src/app/app.component.ts
. You will se the following
content
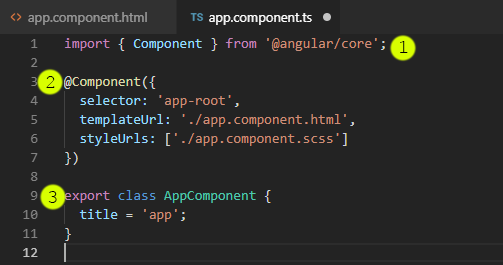
The app.component.ts
file consist of few parts:
1. Imports
At the begining of the component's .ts
file we have to import a Component from the
Angular library. This is required to make our component working. Component is a Decorator
(don't have to bother what does it mean for now) - it provides a configuration metadata that determines how
the component should be processed, initialized and used in a runtime.
Whenever we want to use other components within another component we also have to import it in that place. (we will learn how to do that in a next lesson).
2. Metadata
The @Component
decorator which we imported in a first line allows us to specify a
matadata
for our component. The metadata contains few parameters:
- The
selector
contains the name of the tag that can be used to create this component. - The
templateUrl
contains the relative URL/path to the HTML templatetemplate
(app.component.html
file) - The
styleUrls
contains the array of CSS styles to be used for styling the component or a path to the external file (app.component.scss
file)
You might noticed from a previous lesson, that we have used a <app-root></app-root>
in the index.html
file.
Our component can be called in your HTML code just like any standard HTML tag, i.e:
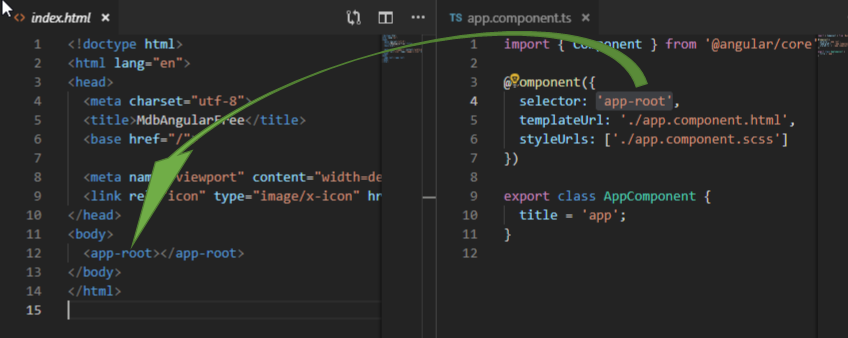
3. Class
The last part of our component is a Component Class which contains atributes and functions. This is the place where entire logic of given component is stored. In a next lesson we will fill this component with some functions to make our application working.
3. Component template (.html)
file)
The template file which is responsible for view of our component. A template looks like regular HTML, except that it also contains Angular template syntax. Our template can use data binding which allows us to synchronize data between our Application and DOM.
4. Component styles (.scss
file)
This file contains all the styles for our component.
5. Component tests (.spec.ts
file)
Within this file we will create a tests of our component. Tests are important for each application, using them help us avoid breaking components logic while making some updates/enhances in it.
6. App.module code (.module.ts
file)
As we mentioned before - Angular application has to contain at least one component. This first component is called a root component.. A root component is the first Angular component that gets bootstrapped when the application runs. Two things are special about this component:
First, if you open the application module file src/app/app.module.ts:
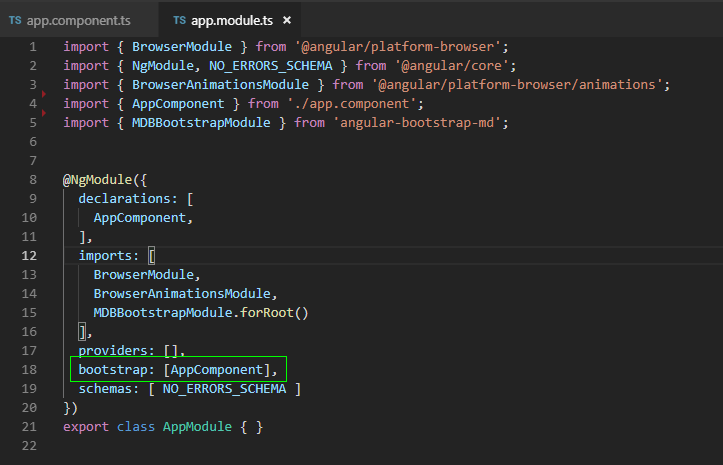
You'll notice that, it's added to the bootstrap array of the module definition.
Second, if you open the src/index.html file (the first file that gets rendered when you visit the application URL) of the application. You'll notice that, it's called inside the document tag.
Important:
app.module.ts
file is only required for our App component (root component). All other
components which we will use inside our application wont require module.ts
file to work.