In this lesson, we will learn how to deal with Inputs. Inputs are very widely used components in web development. We use them everywhere, starting with the login form, newsletter subscription forms, a new post editor through configuration panel etc.
As you seen while playing with the final app, we will use inputs to create a form to add a new event to our daily agenda.
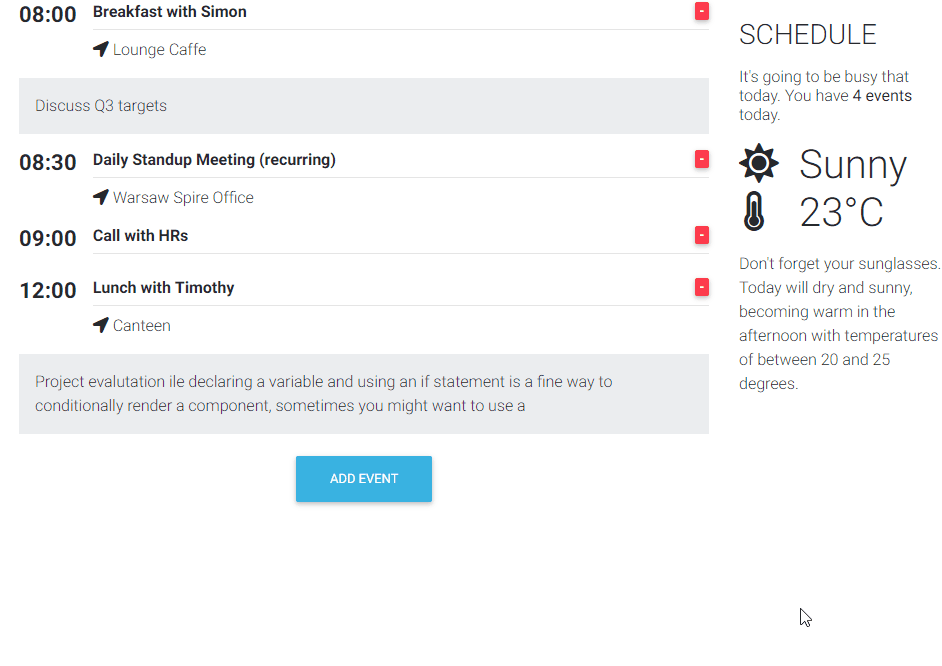
In this lesson we will learn how to deal with forms.
1. ngModel
- Import
FormsModule
inapp.module.ts
: - Add
FormsModule
to the imports[] array: - Add a new variable to
app.component.ts
and call it name - Update the Modal Body within the
app.component.html
template - Save and run the project.
- Add a new function (app.component.ts) and the button (app.component.html) to trigger the function as shown below
- Save the file and run the project
- remove name variable and changeName() function in
app.component.ts
- Empty Modal Body div in
app.component.html
- Import following
ReactiveFormsModule
and add it to imports[] - Import FormControl in
app.component.ts
- Define new variable in
app.component.ts
- Update the Modal Body in
app.component.html
with the following code - Save and run project.
The easiest way to work with forms is to use ngModel
directive. Let's see how it works.

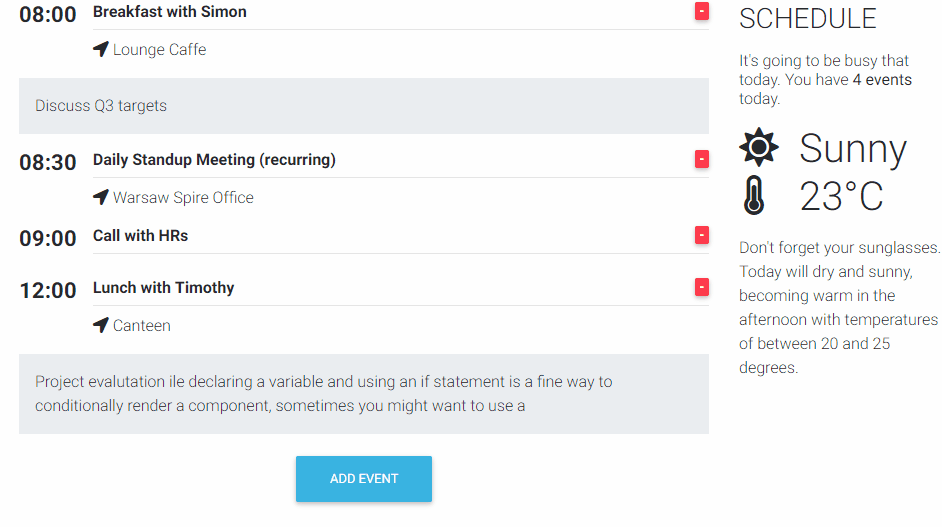
As you can see we have binded our view (Input) with the model (variable) thanks to ngModel
.
Furhter, this works in both ways, that's why it's called two-way Data Bindings.
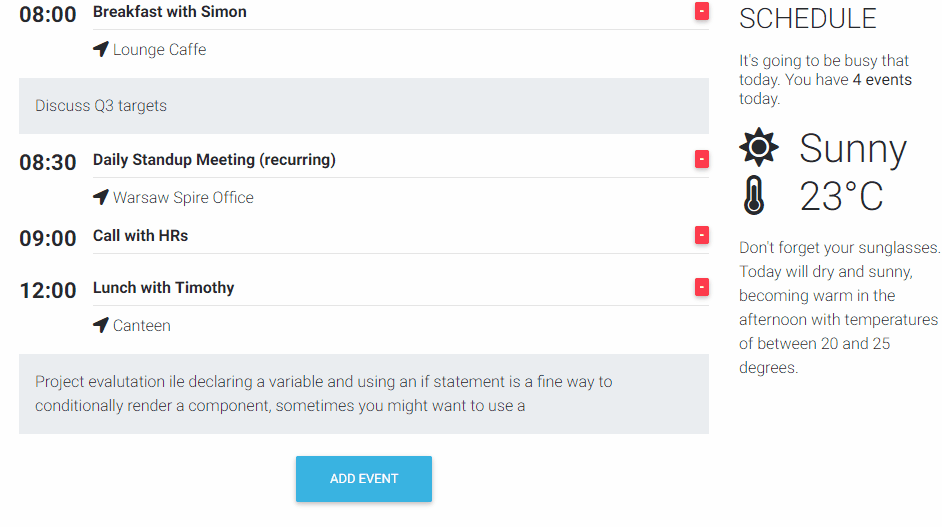
Two-way binding means that any data-related changes affecting the model are immediately propagated to the matching view(s), and that any changes made in the view(s) (say, by the user) are immediately reflected in the underlying model. When app data changes, so does the UI, and conversely.
So ngModel
is the thing in Angular that implements two-way data binding. It’s not the only
thing that does that, but it’s in most cases the directive we want to use for simple scenarios. We will
dedicate a separate lesson to dive into more options.
For now I want you to remember that Angular comes with three different ways of building forms in our applications. There’s the template-driven approach which allows us to build forms with very little to none application code required, then there’s the model-driven or reactive approach using low level APIs, which makes our forms testable without a DOM being required.
The ngModel
is a template-driven case. Now I am gonna show you how to use a
model-driven approach.
Before we move on you can revert the changes:
2. formControl
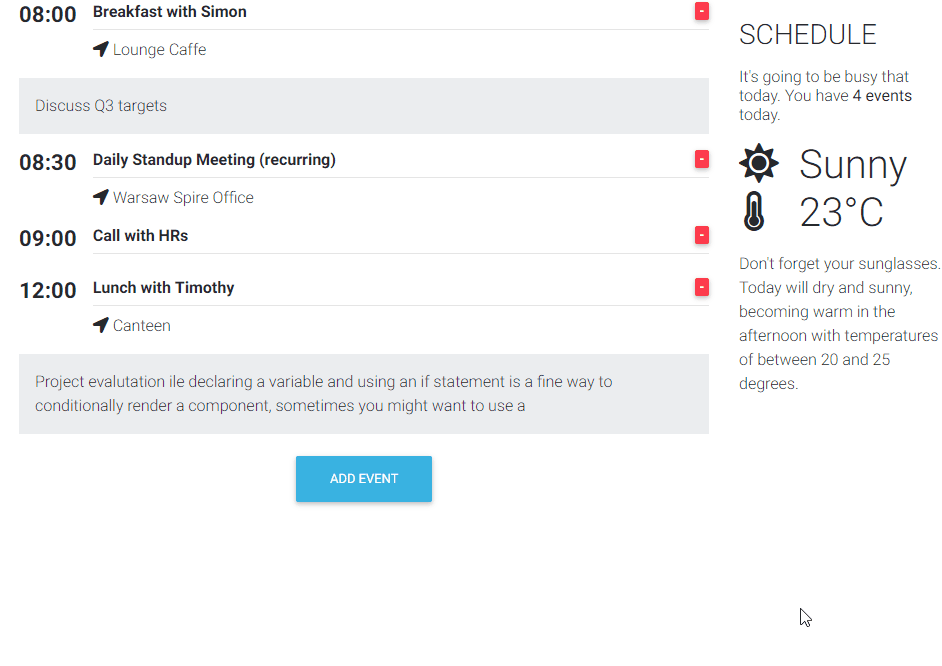