In this lesson, we will learn how to use modals in our projects.
Note:
Modals are small pop up windows which are really handy
when you want to display to user extra content, configuration or consent request.
To use modals, follow the steps below.
- 1. Import the required
ViewChild
andModal
directives inapp.component.ts
- Define the
@ViewChild
element within the AppComponent class - Add a modal template at the end of the
app.component.html
file: - Update the Add event button with modal call:
- Run and test the app
Our modal is almost ready, the last thing we have to do is to display it. In order to do that we will use
frame.show() function (which comes with ModalDirective
) and bind it to the Add
event button using (click) binding.
That how your app.component.html
file should looks like:
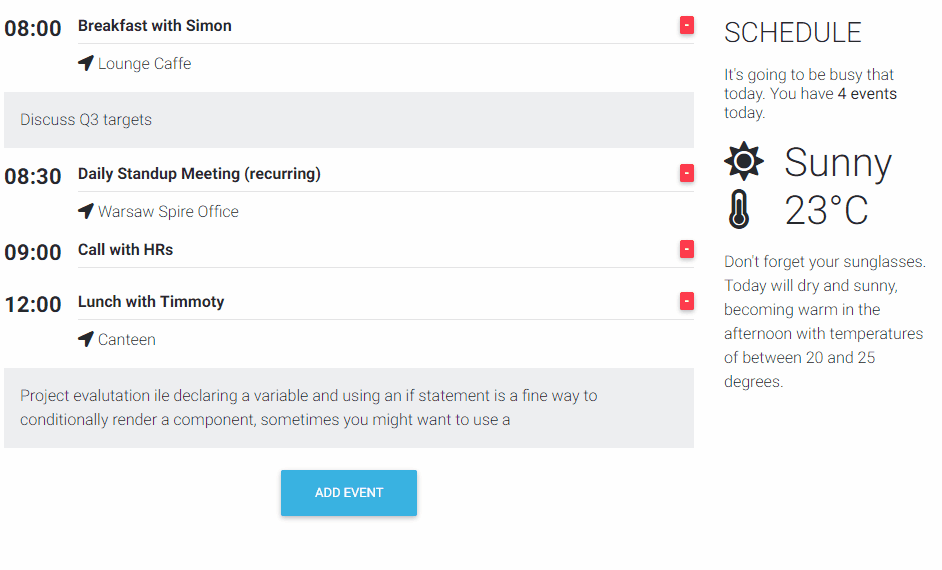
Note:
Modals are great tools and they can be customized in various ways. Most probably you will use them to
get user consent for using cookies or to accept a privacy policy on a website, display a
registration/login form or some extra details like map and/or contact form.
You can find dozens of predefined modal templates in our docs.
You may also wish to check out our modal generator.