Our first TypeScript code
Before you start:
Make sure that you have installed TypeScript — if you haven't check this lesson first.
Let's write our first line of TypeScript code. Before we will do it let me clarify one thing. TypeScript is independent of Angular, therefore you can use TypeScript without using Angular. Let's see how to do that.
- Open a terminal and create a file
main.ts
: - Now run following command:
- Let's run our js code using node:
This will run TypeScript compiler and compile TypeScript to JavaScript. If you run ls
(macOS/unix) or dir
(Windows) command. You should see 2 files:
Our TypeScript code (main.ts) was compiled to JavaScript (main.js).
You should see:
And again step by step:
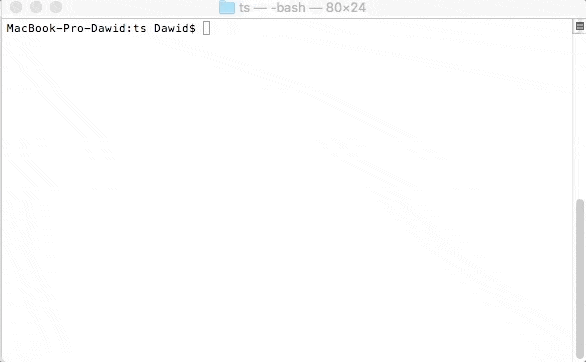
That confirms what we I have stated before:
Any valid JavaScript code is also a valid TypeScript code.
Visual Studio Code
Now you understand that TypeScript is independent of Angular, however, Angular cannot work without TypeScript.
Therefore whenever you are running ng serve
, or ng build
, Angular CLI is calling the
TypeScript compiler under the hood, so to compile the code of our application to JavaScript that can be used by
browsers.
While working with Angular you will hardly use the tsc
command and stand-alone files, therefore we
will switch to Visual Studio Code now.
- Open Visual Studio Code
- Open our recent project
/my-app
(if you missed that check previous tutorial where we set up our project). - Replace the content of
/src/app/app.component.ts
file with the following: - Save the file, then run
ng serve -o
. When the CLI opens a new tab/window with our project, open the developer console. You will see:

As you noticed we have created a function — onStartup()
— and added it to the constructor of our
AppComponent
. Whenever an instance of our component is created, it will call our
onStartup
function.