Fundamentals
In order to create Angular apps, you have to know Typescript. Within thenext few lessons I will teach you its basic concepts, by covering the following subjects:
- Type annotations
- Arrow functions
- Interfaces
- Classes
- Constructors
- Access modifiers
- Properties
- Modules
If you are familiar with these concepts you can skip this tutorial and navigate to the next part, however if you are new to TypeScript then follow it carefully.
What is TypeScript?
At a first glance you might confuse TypeScript with JavaScript. Especially that any valid JavaScript code is also a valid TypeScript code, however, it doesn't work another way around. TypeScript is a superset of JavaScript.
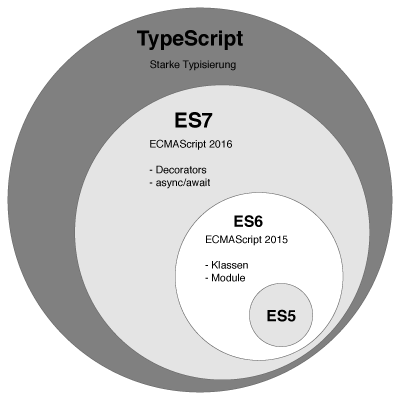
Image taken from https://angular2buch.de
Remember:
TypeScript is a superset of JavaScript
That means that TypeScript extends JavaScript with extra functionality which is not present in the current version of JavaScript supported by most browsers. So what are those features?
Strong typing
If you are familiar with languages like Java or C# you probably know that each variable requires a type and this type has to be declared upfront. JavaScript is a loosely typed or a dynamically typed language. Variables in JavaScript are not directly associated with any particular value type, and any variable can be assigned (and re-assigned) values of all types:
In a weakly typed language, the type of a value depends on how it is used. For example, if I can pass a string to the addition operator and it will AutoMagically be interpreted as a number or cause an error if the contents of the string cannot be translated into a number. Similarly, I can concatenate strings and numbers or use strings as booleans, etc.
In a strongly typed language, a variable has a type and that type cannot change. What you can do to a variable depends on its type. If we try to compile the above code in TypeScript we will get the sfollowing error:
The advantage of a strongly typed language is that you are forced to make the behavior of your program explicit. If you want to add a number and a string your code, you must translate the string into a number to be used as an operand of the addition operator. This makes code easier to understand because there is no (or less) hidden behavior. This also makes your code more verbose.
Remember:
Strong typing is optional in TypeScript but using this feature makes your application more predictable and make it easier to debug so you should definitely make use of it.
Object-oriented
Object-oriented programming (OOP) is an old concept used by multiple languages like Java or C# to help developer
associate together data and methods within one "object". In OOP
object are created and are able to
interact with each other using one another's public facing methods. JavaScript itself is not an
object-oriented language in the way that C++, C#, or Java are. TypeScript, on the other
hand, can be treated as an object-oriented language because of the language constructs it
introduces on top of JavaScript closures.
TypeScript brings many object-oriented features which we missed in JavaScript for a very long time. We have explicit concepts of:
- Classes
- Interfaces
- Constructors
- Access modifiers (public and private)
We will learn all of that in the later sections.
Compile-time error
Let me explain first what run-time error
is as this is a common case. We are talking about the
run-time error
when we face any issue while using our application. In other words — if you make a
mistake when you create your website and write some code, you will see it only while using the
application/website. For example, if you make a typo in an webpage file your browser console will show you an
error when you load that page.
Due to weak typing in JavaScript, it often happens that we try to perform operations on a variable with a different type. If our application logic is complex we might not spot that we are trying to assign elements of a different type to each other. In JavaScript, we will get to know about the error only when someone triggers code and it fails. In contrast, TypeScript can provide us with compile-time errors, which means, it can spot errors while compiling code to JavaScript, and fix it before deploying to production. Of course it won't catch them all, but still a lot.
Awesome tool-set
I won't write much about this. Along with this course you will see how many useful tools come with Angular.
Let's don't waste time and start coding!