In this lesson, we will cover a different type of variables and scopes.
- Replace the content of the
onStartup()
function: - And start our application, what you will see?
- Let's change
var
, tolet
: - Let's have a look at the error which occurred in line 22 after we change the scope of our variable:
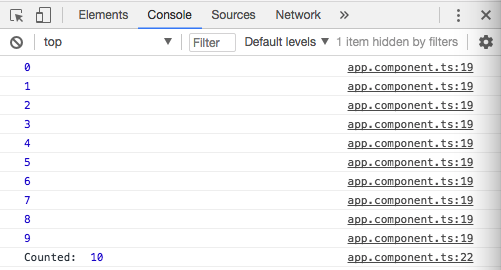
Note that although our i
variable was declared inside a for
loop, it is accessible
to the console.log()
function which is outside of our
code block. Why?
This is because we have declared our variable as var
. In JavaScript, all variables declared like
that have scope to the nearest function, which in our case is count()
.
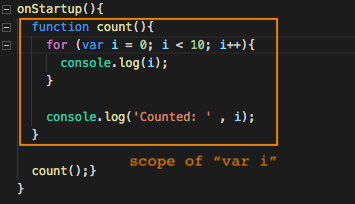
Now the scope of our variable is changed to local:
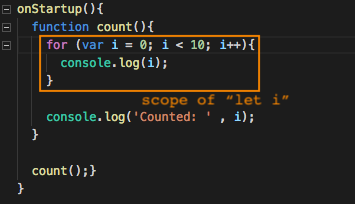
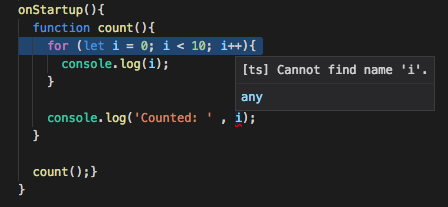
and if we save the file, we will see an error in the console during compilation:
Remember when we talked about compile-time errors? This is a beautiful example, the compiler spots this error and informs us that we are trying to access a variable which isn't accessible within the current scope before we even tried to run our code in a browser. Actually, thanks to Visual Studio we can see an error even before we request the CLI to compile our project. The editor shows us errors in real-time. That's a really awesome feature which will save you a lot of time.