As we learned in a previous lesson, TypeScript allows us to use static typing and use different type of variables. Each variable defines a certain set of functions which can be used along with them. For instance:
- Declare the following variables in
app.components.ts
: - In a new line, type each of the variables, followed by dot (ie.
a.
) and observe the result:
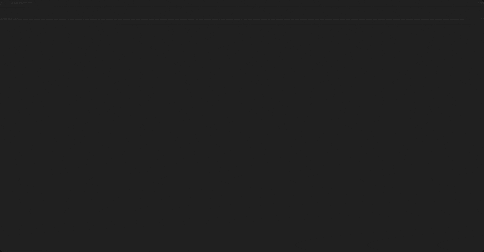
As you may notice, Visual Studio suggests functions which can be used along with each variable type.
Numeric type methods:
# | Method | Description | Result |
---|---|---|---|
1 | a = 5.56789; | Basic assignment | 5.56789 |
2 | a.toExponential() | Convert a number into an exponential notation: | 5.56789e+0 |
3a | a.toFixed() | Converts a number to a string keeping a specified number of decimals. | 6 |
3b | a.toFixed(2) | 5.57 | |
4 | a.toLocaleString('ar-EG') | Returns a string with a language sensitive representation of this number. | ٥٫٥٦٨ |
5 | a.toPrecision(2) | Returns a string representing the Number object to a specified precision. While toFixed(n) provides n length after the decimal point; toPrecision(x) provides x total length. |
5.6 |
6 | a.toString() | Returns a string representing the Number object. | 5.56789 |
7 | a.valueOf() | The valueOf() method returns the wrapped primitive value of a Number object. | 5.56789 |
String type methods:
Warning
The string prototype in TypeScript offers more default methods than described below, however, they are marked as
deprecated
. That means, that they will be removed in the future so you should avoid using them. Some browsers may still support them, though.
# | Method | Description | Result |
---|---|---|---|
1 | b = 'Hello World'; | Basic assignment | Hello World |
2 | b.charAt(6) | The String object's charAt() method returns a new string consisting of the single UTF-16 code unit located at the specified offset into the string. | M |
3a | b.charCodeAt(6) | The charCodeAt() method returns an integer between 0 and 65535 representing the UTF-16 code unit at the given index. | 77 |
3b | b.concat('. It is nice to meet you.'); | The concat() method combines the text of one or more strings and returns a new string. | Hello Wolrd. It is nice to meet you. |
4 | b.endsWith('d') | The endsWith() method determines whether a string ends with the characters of a specified string, returning true or false as appropriate. | true |
5.1 | b.includes('B'); | The includes() method determines whether one string may be found within another string, returning true or false as appropriate. | true |
5.2 | b.includes('b'); | false | |
6 | a.indexOf('M') | The indexOf() method returns the index within the calling String object of the first occurrence of the specified value, starting the search at fromIndex. Returns -1 if the value is not found. | 6 |
7 | a.lastIndexOf('l') | The lastIndexOf() method returns the index within the calling String object of the last occurrence of the specified value, searching backwards from fromIndex. Returns -1 if the value is not found. | 12 |
Coming soon:
- b.localeCompare()
- b.match()
- b.normalize()
- b.padEnd()
- b.padStart()
- b.repeat()
- b.replace()
- b.search()
- b.slice()
- b.split()
- b.startsWith()
- b.substr()
- b.substring()
- b.toLocaleLowerCase()
- b.toLocaleUpperCase()
- b.toLowerCase()
- b.toString()
- b.toUpperCase()
- b.trim()
- b.trimLeft()
- b.trimRight()
- b.valueOf()
- b.[@@iterator]()
- b.raw()
Any and boolean
The any type doesn't offer any predefined methods. Boolean offers two basic methods — toString
which
returns a string of either 'true' or 'false, as well as valueOf
which returns the primitive
value
of the Boolean object.